API Details (no longer supported/maintained)
Note: API currently uses Basic Authentication. Make API calls using https only.
Common live working example of all the API calls is provided at the bottom.
And there will be more settings getting added in the future, so try to make it a bit flexible.
Editing individual slot Settings:
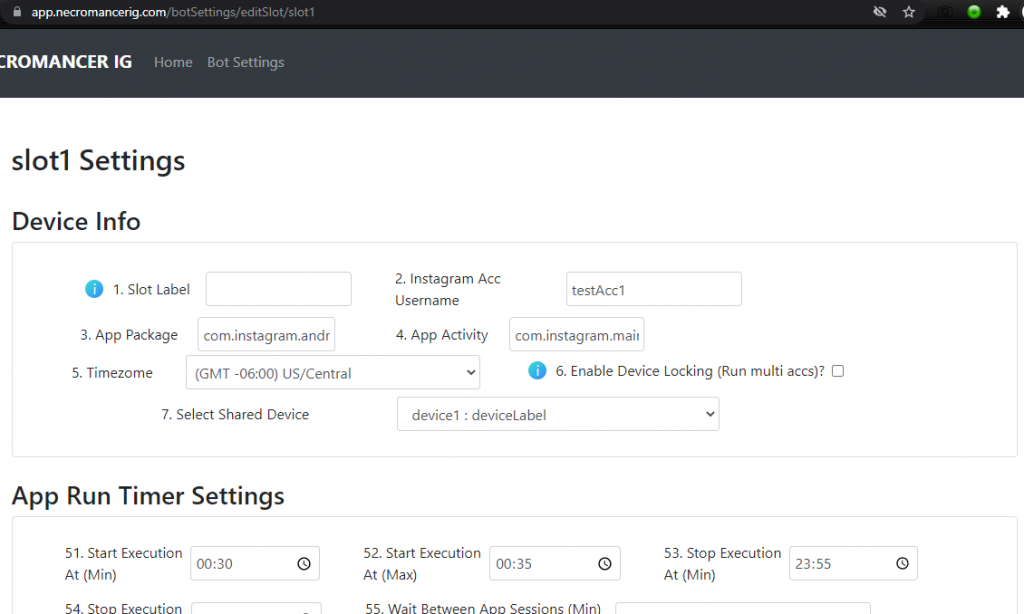
Make a GET request on https://app.necromancerig.com/api/e/getSlotSettings/{slotId} for getting the slot details Settings in a JSON format.
Make a POST request on https://app.necromancerig.com/api/e/setSlotSettings/{slotId} for setting the slot details Settings. (And the request content should be all the settings in the JSON format/object.)
Editing individual slot Target Data:
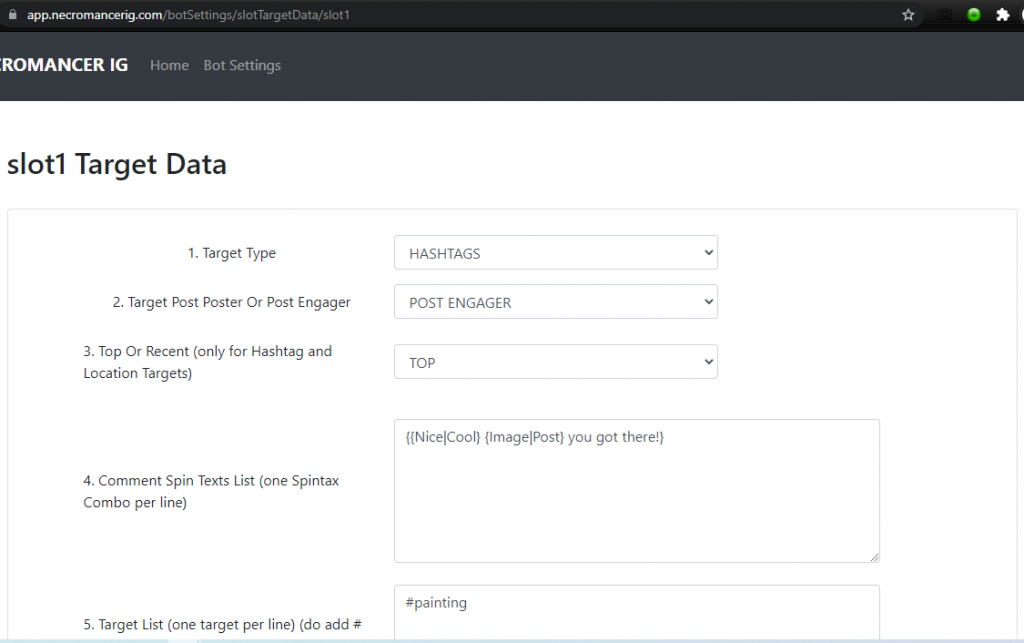
Make a GET request on https://app.necromancerig.com/api/e/getSlotTargetData/{slotId} for getting the slot target data in a JSON format.
Make a POST request on https://app.necromancerig.com/api/e/setSlotTargetData/{slotId} for setting the slot target data. (And the request content should be all the settings in the JSON format/object.)
Getting individual slot Sats:

Make a GET request on https://app.necromancerig.com/api/e/getSlotStats/{slotId} for getting the slot stats in a JSON format.
Editing individual device Settings:

Make a GET request on https://app.necromancerig.com/api/e/getDeviceSettings/{deviceId} for getting the device Settings in a JSON format.
Make a POST request on https://app.necromancerig.com/api/e/setDeviceSettings/{deviceId} for setting the device Settings. (And the request content should be all the settings in the JSON format/object.)
Getting Main Dashboard Mini Infos:
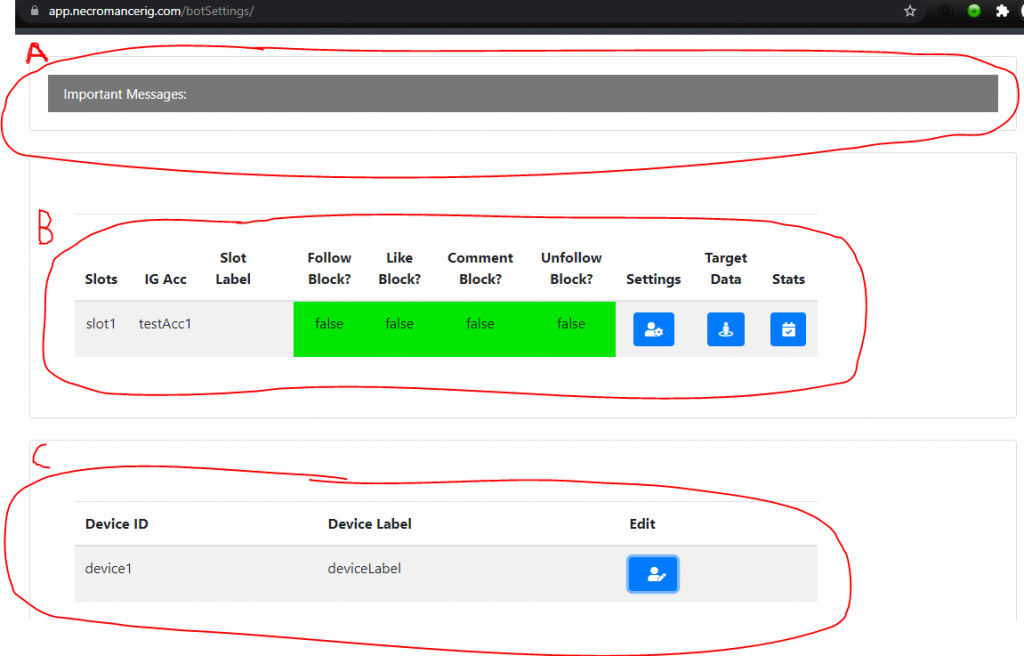
For getting “A” (/Important Messages), Make a GET request on https://app.necromancerig.com/api/e/getCriticalMessages/
For getting “B” (/all slots mini infos), Make a GET request on https://app.necromancerig.com/api/e/getMainDashboardSlotsInfo/
For getting “C” (/all devices info), Make a GET request on https://app.necromancerig.com/api/e/getMainDashboardAllDevices/
Common Online Live Working Example of all the above API calls:
1st Goto browxy.com
And then do the below steps to see the live working Example.

# Image 1 Steps:
1) Select “Java – Maven” from the Dropdown.
2) Click on “Open Example” Button.
3) Remove Everything from there, and paste this below code in its place. (And press “Cntrl+S” for saving it.)
package domain;
import kong.unirest.HttpResponse;
import kong.unirest.JsonNode;
import kong.unirest.Unirest;
import kong.unirest.json.JSONArray;
import kong.unirest.json.JSONObject;
import java.nio.charset.StandardCharsets;
import java.util.Base64;
public class HelloWorld {
public static void main(String[] args) {
String userName = “tempemail@gmail.com”, password = “Asdf12!@”;
String loginString = userName + “:” + password;
String encodedCreds = Base64.getEncoder().encodeToString(loginString.getBytes(StandardCharsets.UTF_8));
String baseUrl = “https://app.necromancerig.com”;
String slotId = “slot1”, deviceId = “device1”;
System.out.println(“\n\n\n ======= Started ======= \n”);
//======================================= API DETAILS EXAMPLES =============================================================
//================== Slot Settings =============================
//Getting Slot Settings JSON
HttpResponse<JsonNode> getSlotSettingsJsonResponse = Unirest.get(baseUrl+”/api/e/getSlotSettings/”+slotId)
.header(“Authorization”, “Basic ” + encodedCreds).asJson();
System.out.println(“\n getSlotSettingsJsonResponse: ” + getSlotSettingsJsonResponse.getBody());
//Modifying Slot Setting object for demonstrating API post below
JSONObject slotSettingsJsonToSend = getSlotSettingsJsonResponse.getBody().getObject();
slotSettingsJsonToSend.put(“slotLabel”, “wohooX5”); //can test modifying any value here, for testing the API post.
//Sending Slot Settings JSON for saving
HttpResponse<JsonNode> jsonResponse = Unirest.post(baseUrl+”/api/e/setSlotSettings/”+slotId)
.header(“Authorization”, “Basic ” + encodedCreds)
.header(“Content-Type”, “application/json”)
.header(“accept”, “application/json”)
.body(slotSettingsJsonToSend).asJson();
//================== Slot Target Data =============================
//Getting Slot Target Data JSON
HttpResponse<JsonNode> getSlotTargetDataJsonResponse = Unirest.get(baseUrl+”/api/e/getSlotTargetData/”+slotId)
.header(“Authorization”, “Basic ” + encodedCreds).asJson();
System.out.println(“\n getSlotTargetDataJsonResponse: ” + getSlotTargetDataJsonResponse.getBody());
//Modifying Slot Target Data object for demonstrating API post below
JSONObject slotTargetDataJsonToSend = getSlotTargetDataJsonResponse.getBody().getObject();
slotTargetDataJsonToSend.put(“commentSpinTextsList”, “{Hi|HelloX5}”); //can test modifying any value here, for testing the API post.
//Sending Slot Target Data JSON for saving
HttpResponse<JsonNode> slotTargetDataJsonResponse = Unirest.post(baseUrl+”/api/e/setSlotTargetData/”+slotId)
.header(“Authorization”, “Basic ” + encodedCreds)
.header(“Content-Type”, “application/json”)
.header(“accept”, “application/json”)
.body(slotTargetDataJsonToSend).asJson();
//====================== Slot Stats ==============================
//Getting Slot Stats JSON
HttpResponse<JsonNode> getSlotStatsJsonResponse = Unirest.get(baseUrl+”/api/e/getSlotStats/”+slotId)
.header(“Authorization”, “Basic ” + encodedCreds).asJson();
System.out.println(“\n getSlotStatsJsonResponse: ” + getSlotStatsJsonResponse.getBody());
//=================== Device Settings =============================
//Getting Device Settings JSON
HttpResponse<JsonNode> getDeviceSettingsJsonResponse = Unirest.get(baseUrl+”/api/e/getDeviceSettings/”+deviceId)
.header(“Authorization”, “Basic ” + encodedCreds).asJson();
System.out.println(“\n getDeviceSettingsJsonResponse: ” + getDeviceSettingsJsonResponse.getBody());
//Modifying Device Setting object for demonstrating API post below
JSONObject deviceSettingsJsonToSend = getDeviceSettingsJsonResponse.getBody().getObject();
deviceSettingsJsonToSend.put(“deviceLabel”, “wohooX5”); //can test modifying any value here, for testing the API post.
//Sending Device Settings JSON for saving
HttpResponse<JsonNode> deviceSettingsJsonResponse = Unirest.post(baseUrl+”/api/e/setDeviceSettings/”+deviceId)
.header(“Authorization”, “Basic ” + encodedCreds)
.header(“Content-Type”, “application/json”)
.header(“accept”, “application/json”)
.body(deviceSettingsJsonToSend).asJson();
//================ Main DashBoard details ==========================
//Getting Main DashBoard Critical Messages Info
HttpResponse<JsonNode> getCriticalMessagesJsonResponse = Unirest.get(baseUrl+”/api/e/getCriticalMessages/”)
.header(“Authorization”, “Basic ” + encodedCreds).asJson();
System.out.println(“\n getCriticalMessagesJsonResponse: ” + getCriticalMessagesJsonResponse.getBody());
//Getting Main DashBoard Slots Info
HttpResponse<JsonNode> getMainDashboardSlotsInfoJsonResponse = Unirest.get(baseUrl+”/api/e/getMainDashboardSlotsInfo/”)
.header(“Authorization”, “Basic ” + encodedCreds).asJson();
System.out.println(“\n getMainDashboardSlotsInfoJsonResponse: ” + getMainDashboardSlotsInfoJsonResponse.getBody());
//Getting Main DashBoard All Devices Info
HttpResponse<JsonNode> getMainDashboardAllDevicesJsonResponse = Unirest.get(baseUrl+”/api/e/getMainDashboardAllDevices/”)
.header(“Authorization”, “Basic ” + encodedCreds).asJson();
System.out.println(“\n getMainDashboardAllDevicesJsonResponse: ” + getMainDashboardAllDevicesJsonResponse.getBody());
}
}
4) Double click on “pom.xml”

# Image 2 Steps:
1) Paste this below text inbetween those <dependencies> and </dependencies> tags. (And press “Cntrl+S” for saving it.)
<dependency>
<groupId>com.konghq</groupId>
<artifactId>unirest-java</artifactId>
<version>3.7.04</version>
</dependency>
2) Click on this “x” Mark to close this file.
3) And that’s it. We’re done. Now just click on this “Run” Button to run this test program.
Extra Usage Tips:
If you want to check which JSON object element Id is matched to which field on the website,
Then just “Right Click” on the website page, click on “View page source”.

Press “Cntrl+F” to open search window, and search your Json Object element Id (“slotLabel” in the image below).
And you’ll find the Json Object element on the website.
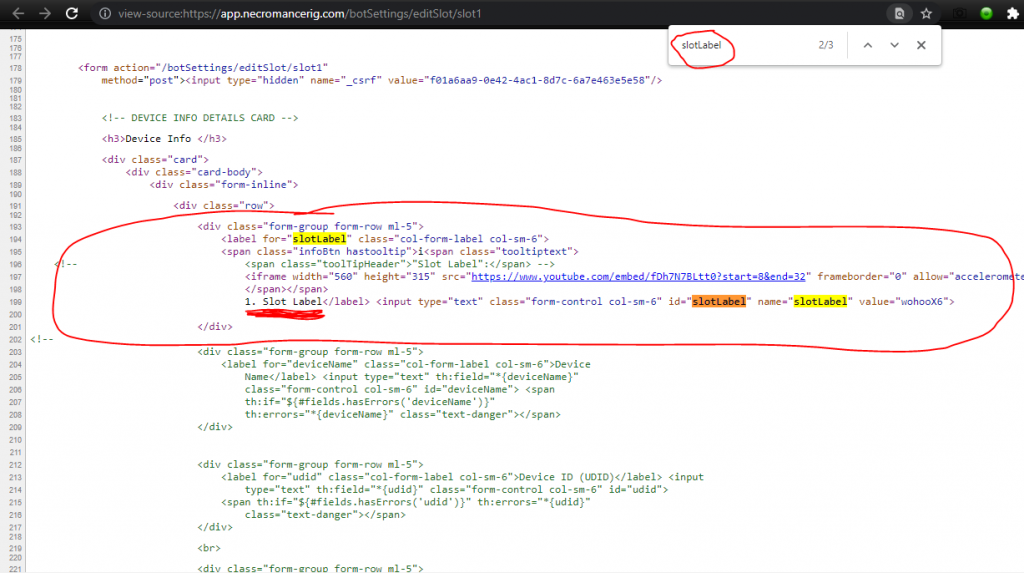
Attached below are the formatted original Json Object originator files (Could be useful during your programming).
Slot Details Json Originator: https://www.dropbox.com/s/ydg8azfh2kyvdg1/ApiSlotDetailsDto.java?dl=1
Slot Target Data Json Originator: https://www.dropbox.com/s/1s47xa9hk57py2x/ApiTargetDataDto.java?dl=1
Slot Stats Json Originator: https://www.dropbox.com/s/xjy0241pdfa5ua4/ApiSlotStatsDto.java?dl=1
Device Settings Json Originator: https://www.dropbox.com/s/5osndu70dqvqxs5/ApiDeviceDto.java?dl=1